Java Interview Questions and Answers
Java is a widely used programming language that has been around for over two decades. It is used in many industries, including finance, healthcare, and gaming, among others.
If you’re looking to land a job as a Java developer, you’ll need to be well-prepared for the interview process.
To help you prepare, we have compiled a list of the most commonly asked Java interview questions and answers. This PDF contains 100 Q&A that cover topics such as Java basics, object-oriented programming, collections, multithreading, exception handling, and more.
Java Interview Questions PDF
By studying these questions and answers, you’ll be well-equipped to tackle any Java interview with confidence. So, let’s dive in and start preparing for your next Java interview!
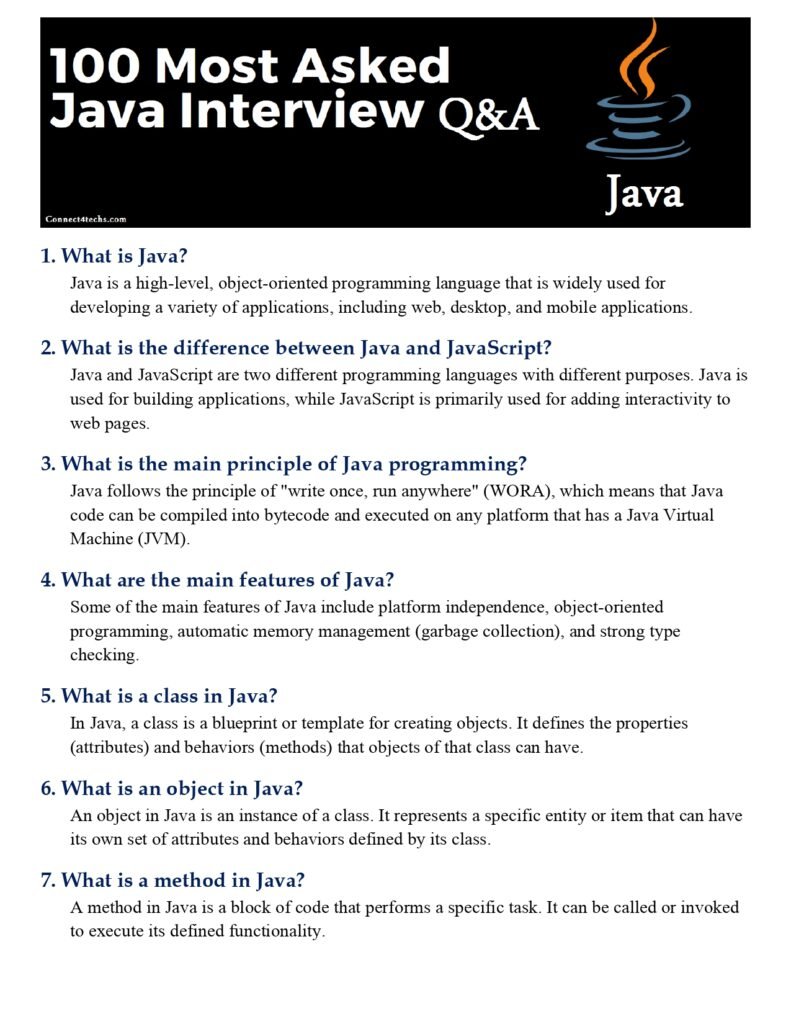
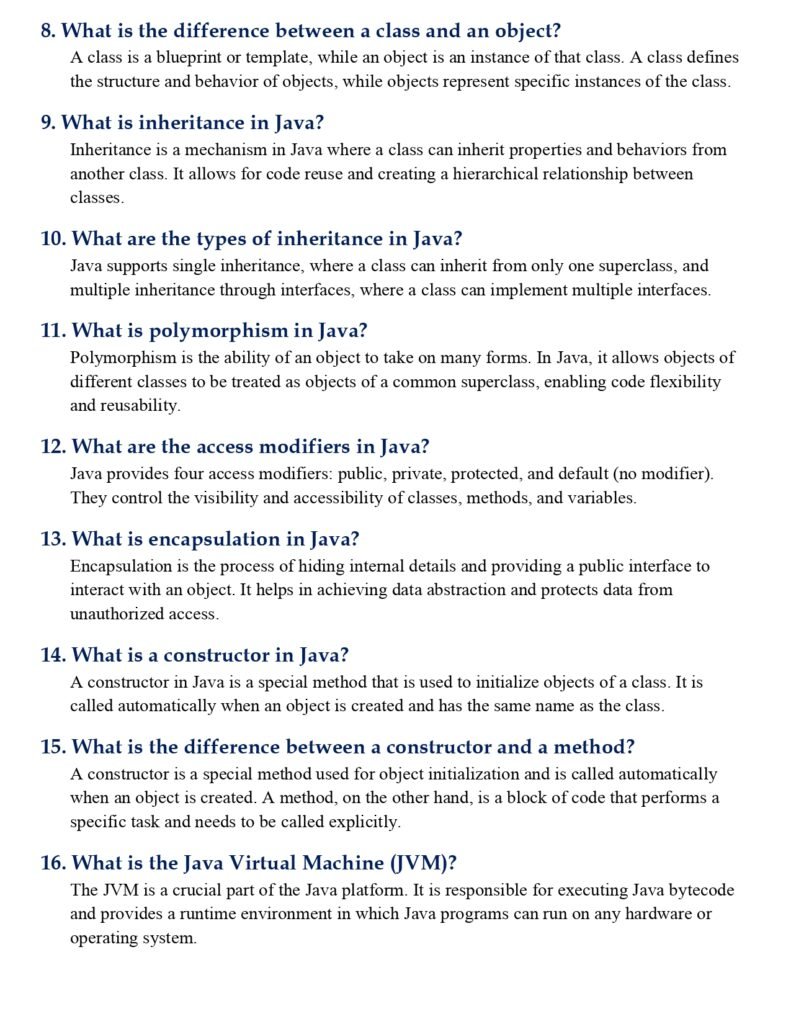
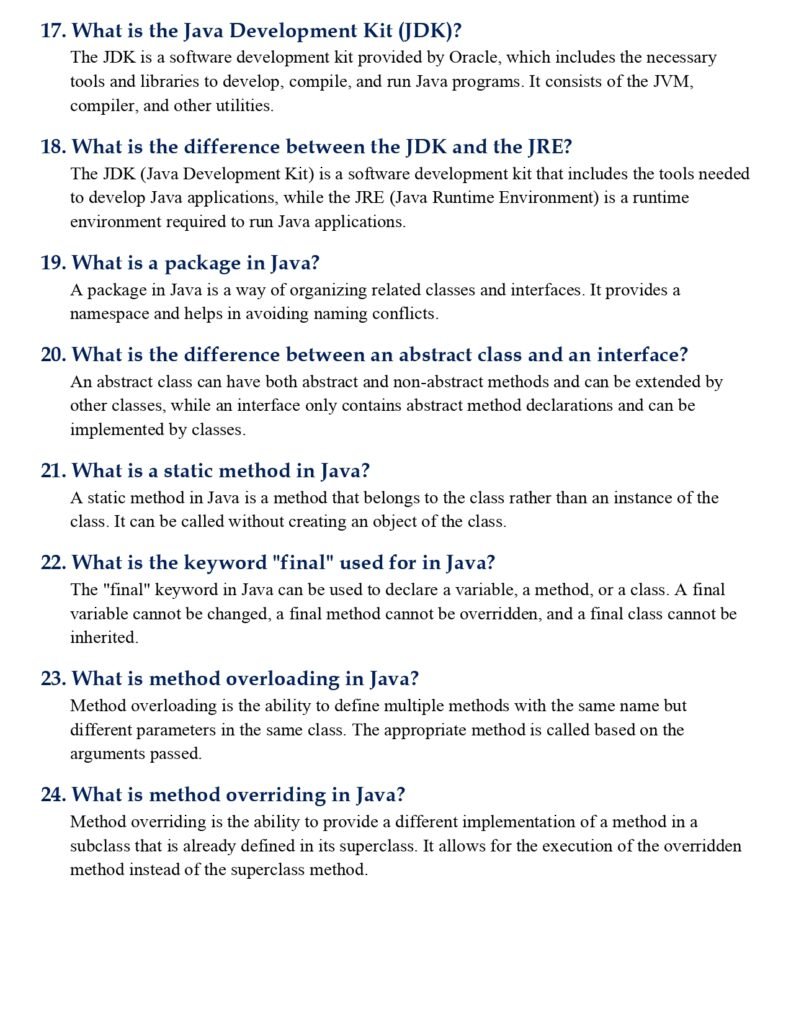
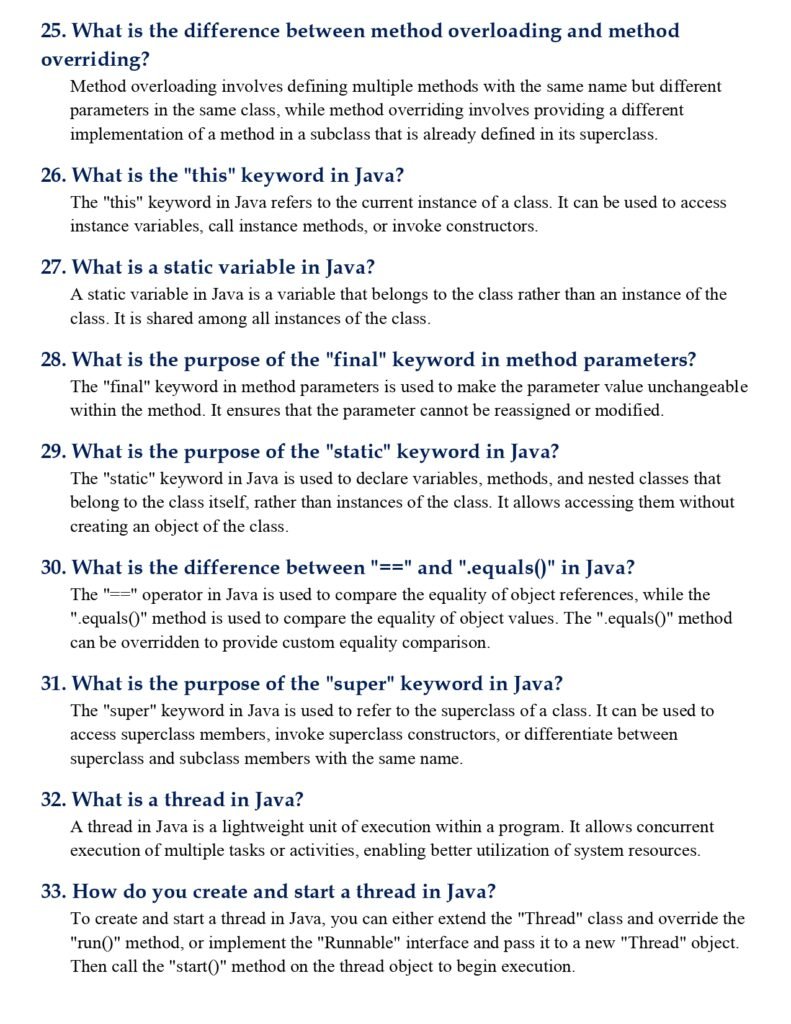
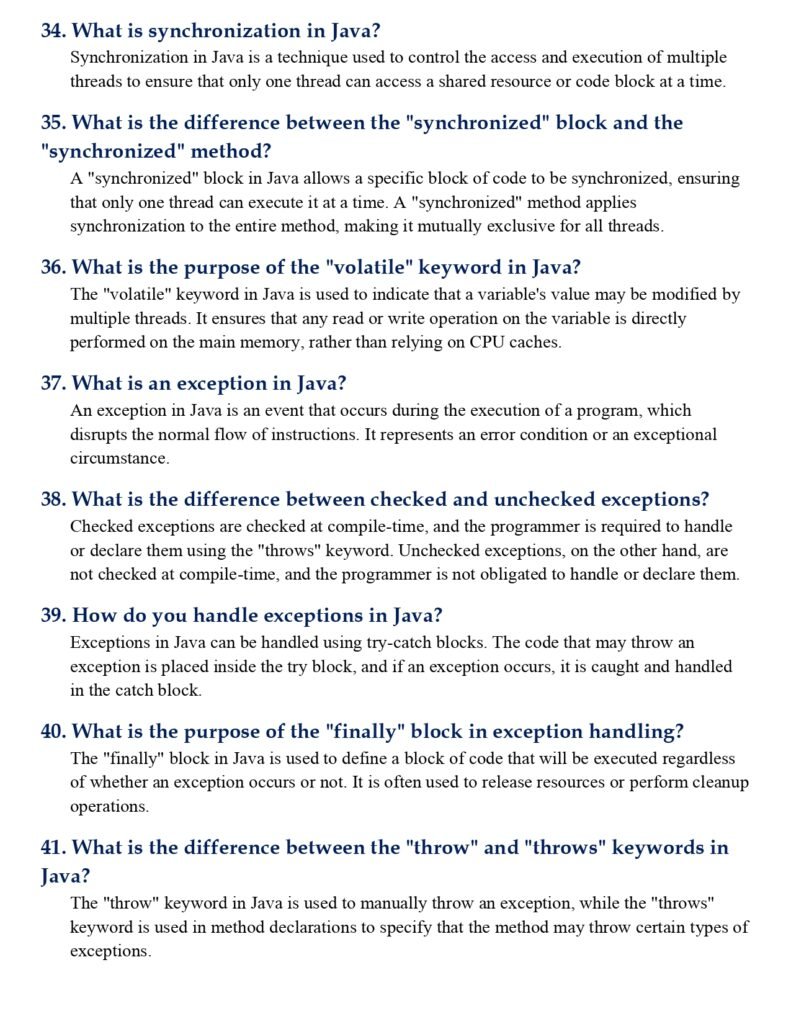
The Contents
- What is Java?
- What is the difference between Java and JavaScript?
- What is the main principle of Java programming?
- What are the main features of Java?
- What is a class in Java?
- What is an object in Java?
- What is a method in Java?
- What is the difference between a class and an object?
- What is inheritance in Java?
- What are the types of inheritance in Java?
- What is polymorphism in Java?
- What are the access modifiers in Java?
- What is encapsulation in Java?
- What is a constructor in Java?
- What is the difference between a constructor and a method?
- What is the Java Virtual Machine (JVM)?
- What is the Java Development Kit (JDK)?
- What is the difference between the JDK and the JRE?
- What is a package in Java?
- What is the difference between an abstract class and an interface?
- What is a static method in Java?
- What is the keyword “final” used for in Java?
- What is method overloading in Java?
- What is method overriding in Java?
- What is the difference between method overloading and method overriding?
- What is the “this” keyword in Java?
- What is a static variable in Java?
- What is the purpose of the “final” keyword in method parameters?
- What is the purpose of the “static” keyword in Java?
- What is the difference between “==” and “.equals()” in Java?
- What is the purpose of the “super” keyword in Java?
- What is a thread in Java?
- How do you create and start a thread in Java?
- What is synchronization in Java?
- What is the difference between the “synchronized” block and the “synchronized” method?
- What is the purpose of the “volatile” keyword in Java?
- What is an exception in Java?
- What is the difference between checked and unchecked exceptions?
- How do you handle exceptions in Java?
- What is the purpose of the “finally” block in exception handling?
- What is the difference between the “throw” and “throws” keywords in Java?
- What is the difference between checked exceptions and runtime exceptions?
- What is the Java API?
- What is the difference between an ArrayList and a LinkedList?
- What is the difference between a HashSet and a TreeSet?
- What is the difference between the “equals()” method and the “hashCode()” method?
- What is the difference between a shallow copy and a deep copy?
- What is a lambda expression in Java?
- What is functional programming in Java?
- What are the Java 8 features for functional programming?
- What is the difference between an interface and an abstract class?
- What is the purpose of the “default” keyword in interface methods?
- What is the difference between a BufferedReader and a Scanner?
- What is the purpose of the “StringBuilder” class in Java?
- What is the difference between the “Comparable” and “Comparator” interfaces?
- What is the purpose of the “assert” keyword in Java?
- What is the difference between a local variable and an instance variable?
- What is the purpose of the “transient” keyword in Java?
- What is the purpose of the “static” block in Java?
- What is the purpose of the “strictfp” keyword in Java?
- What is the difference between a public class and a default (package-private) class?
- What is the purpose of the “enum” keyword in Java?
- What is the purpose of the “break” and “continue” statements in Java?
- What is the purpose of the “try-with-resources” statement in Java?
- What is the purpose of the “instanceof” operator in Java?
- What is the difference between the pre-increment and post-increment operators?
- What is the difference between the pre-decrement and post-decrement operators?
- What is the purpose of the “Math” class in Java?
- What is the purpose of the “StringBuffer” class in Java?
- What is the purpose of the “Math.random()” method in Java?
- What is the purpose of the “Character” class in Java?
- What is the purpose of the “Integer” class in Java?
- What is the purpose of the “Double” class in Java?
- What is the purpose of the “System” class in Java?
- What is the purpose of the “File” class in Java?
- What is the purpose of the “FileNotFoundException” in Java?
- What is the purpose of the “NullPointerException” in Java?
- What is the purpose of the “ArrayIndexOutOfBoundsException” in Java?
- What is the purpose of the “ArithmeticException” in Java?
- What is the purpose of the “NumberFormatException” in Java?
- What is the purpose of the “StringBuilder” class in Java?
- What is the purpose of the “HashSet” class in Java?
- What is the purpose of the “HashMap” class in Java?
- What is the purpose of the “LinkedList” class in Java?
- What is the purpose of the “Comparator” interface in Java?
- What is the purpose of the “Comparable” interface in Java?
- What is the purpose of the “super” keyword in Java?
- What is the purpose of the “this” keyword in Java?
- What is the purpose of the “final” keyword in Java?
- What is the purpose of the “static” keyword in Java?
- What is the purpose of the “abstract” keyword in Java?
- What is the purpose of the “interface” keyword in Java?
- What is the purpose of the “package” keyword in Java?
- What is the purpose of the “import” keyword in Java?
- What is the purpose of the “throw” keyword in Java?
- What is the purpose of the “throws” keyword in Java?
- What is the purpose of the “try-catch-finally” block in Java?
- What is the purpose of the “instanceof” operator in Java?
- What is the purpose of the “break” statement in Java?
- What is the purpose of the “continue” statement in Java?
Nu Of Pages
13 Pages