Welcome to the fourteenth lesson of the “Python From Scratch” series! In this lesson, we will be exploring two important concepts in Python programming – inheritance and iterators.
We will begin by learning about inheritance, which is a fundamental concept in object-oriented programming. Inheritance allows us to create new classes that are based on existing classes, inheriting their properties and methods. We will learn how to use inheritance in Python to create more complex programs with less redundancy.
Next, we will move on to iterators, which are objects that allow us to traverse through a collection of data one element at a time. We will learn how to create iterators in Python, and how to use them to traverse through different types of sequences such as lists, tuples, and dictionaries.
Throughout this lesson, we will be building on the knowledge and skills that we have developed in previous lessons, so it is recommended that you have a solid understanding of Python fundamentals before diving into this one. However, even if you are new to Python, you should be able to follow along with the examples and exercises provided.
Overview about Our Python Lesson 14
Python Inheritance & Iterators Five pages of summary in PDF format to start learning the Python language with a series of lessons that we will complete together to master the Python language
By the end of this lesson, you will have a solid understanding of how to use inheritance and iterators in Python and how to incorporate them into your own programs. So, let’s get started!
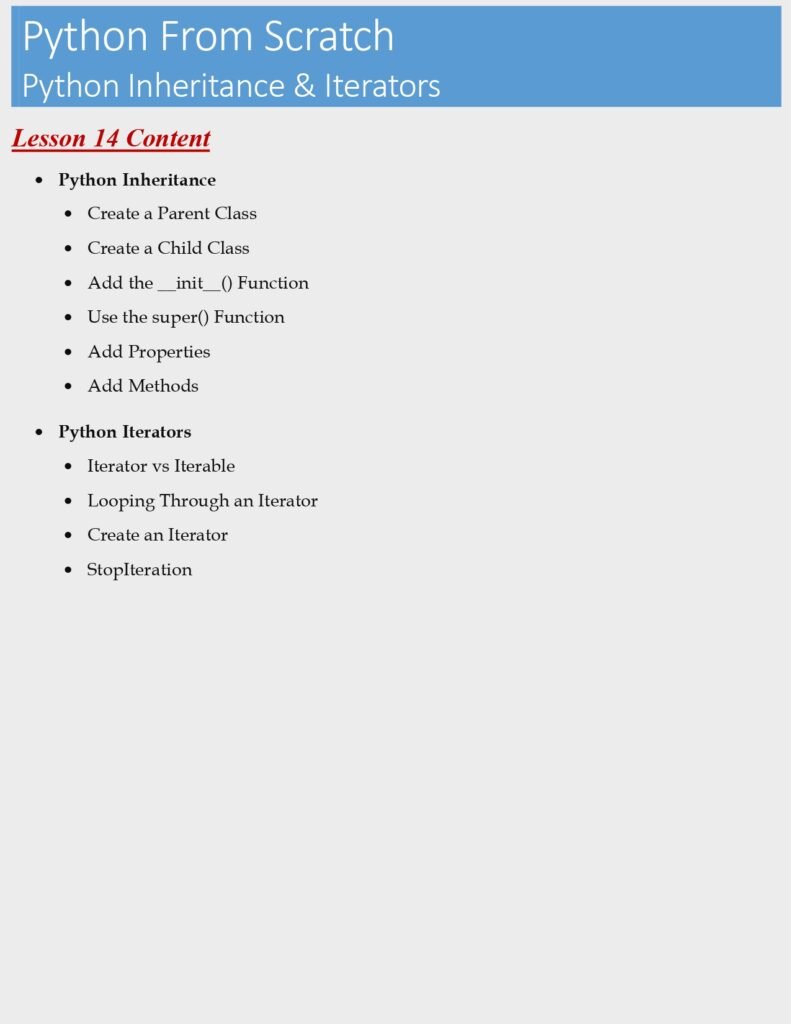
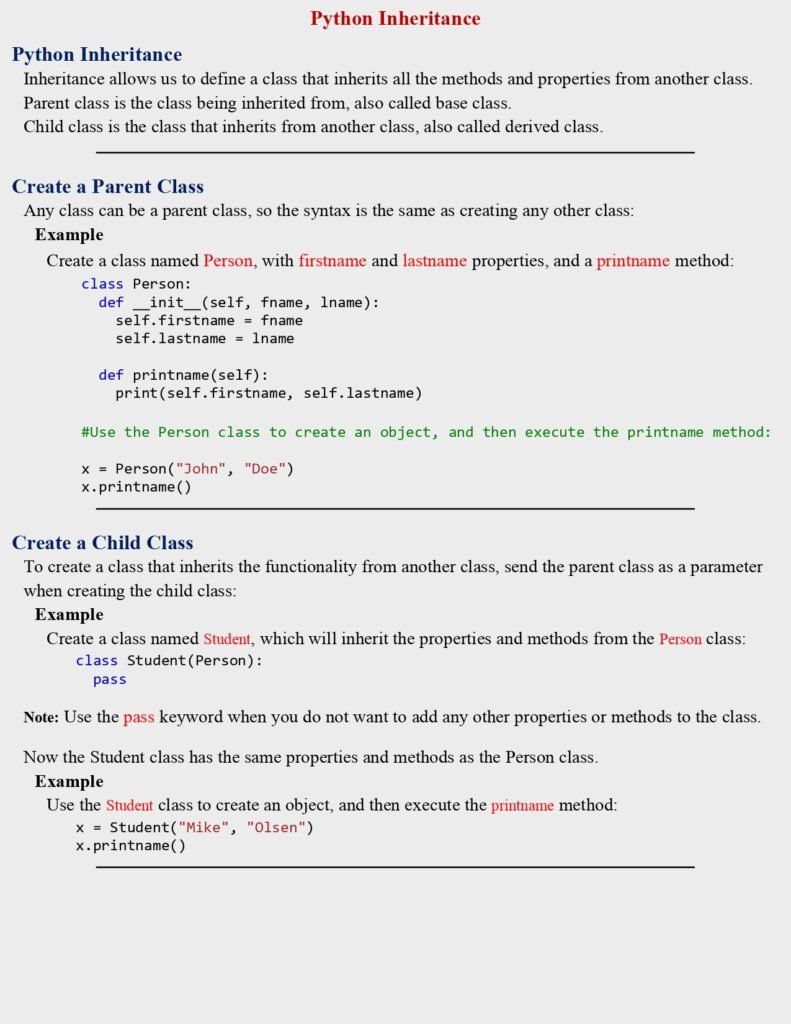
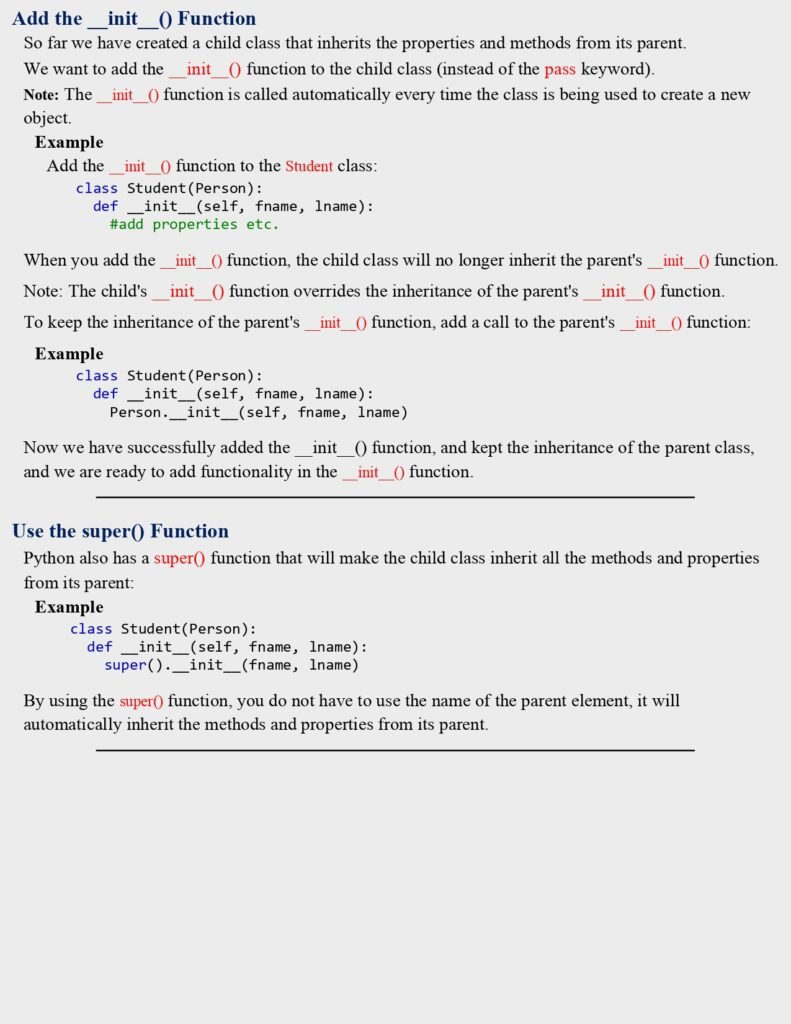
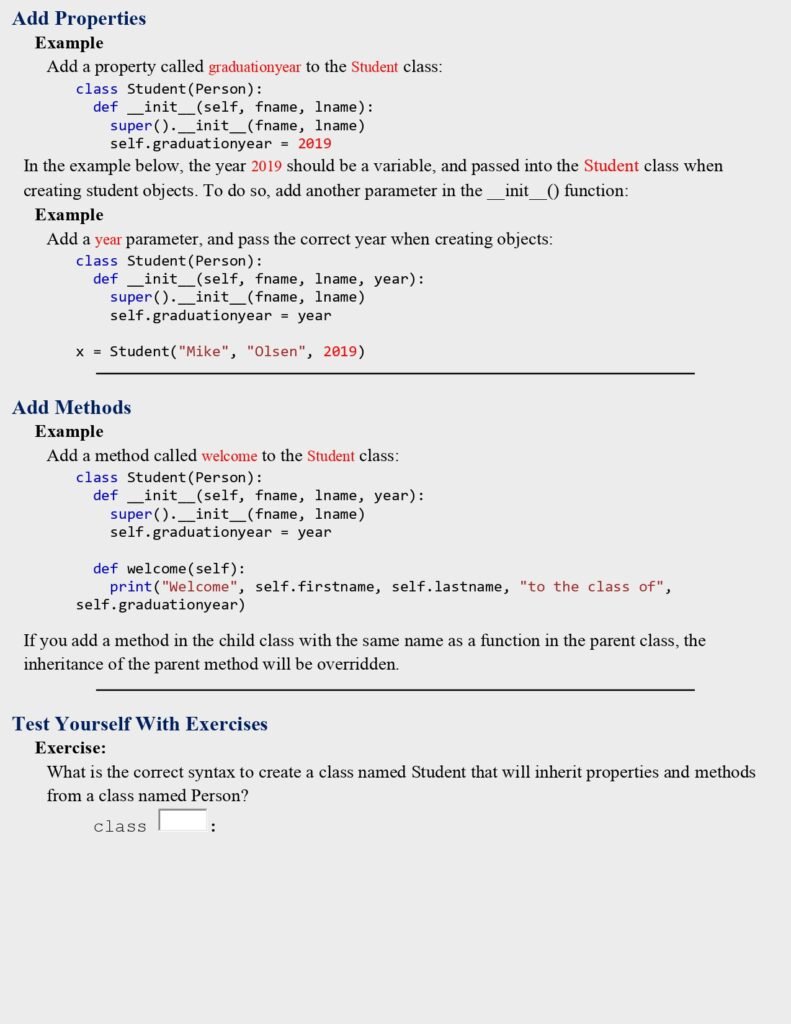
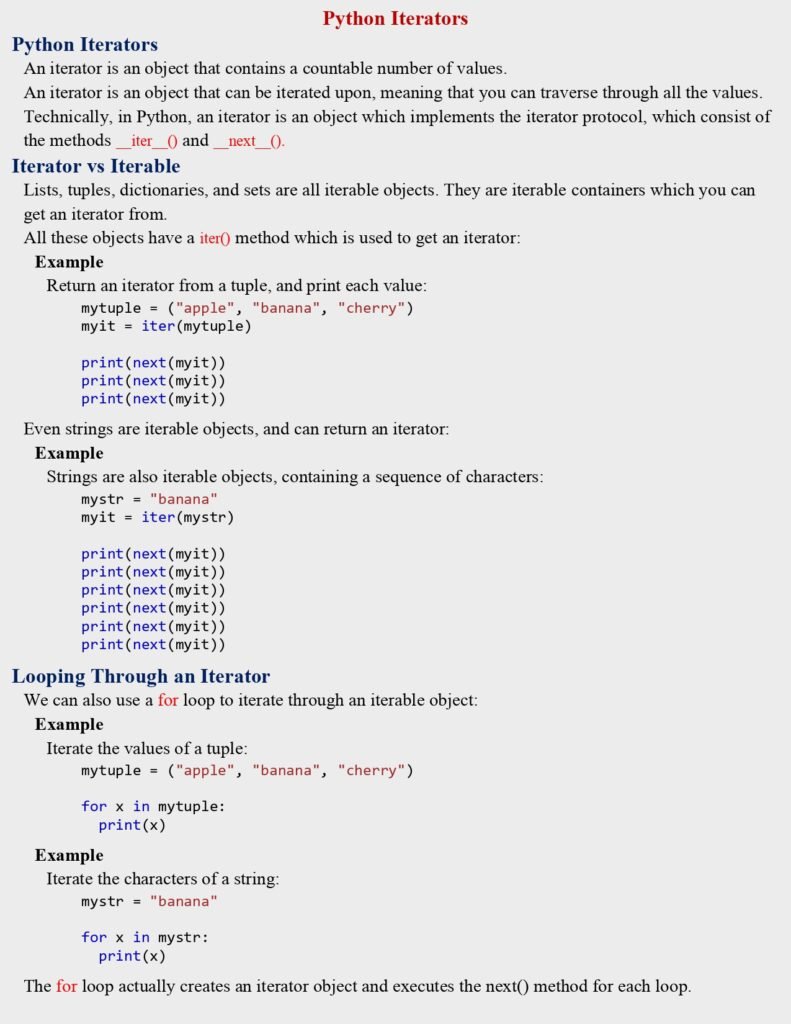
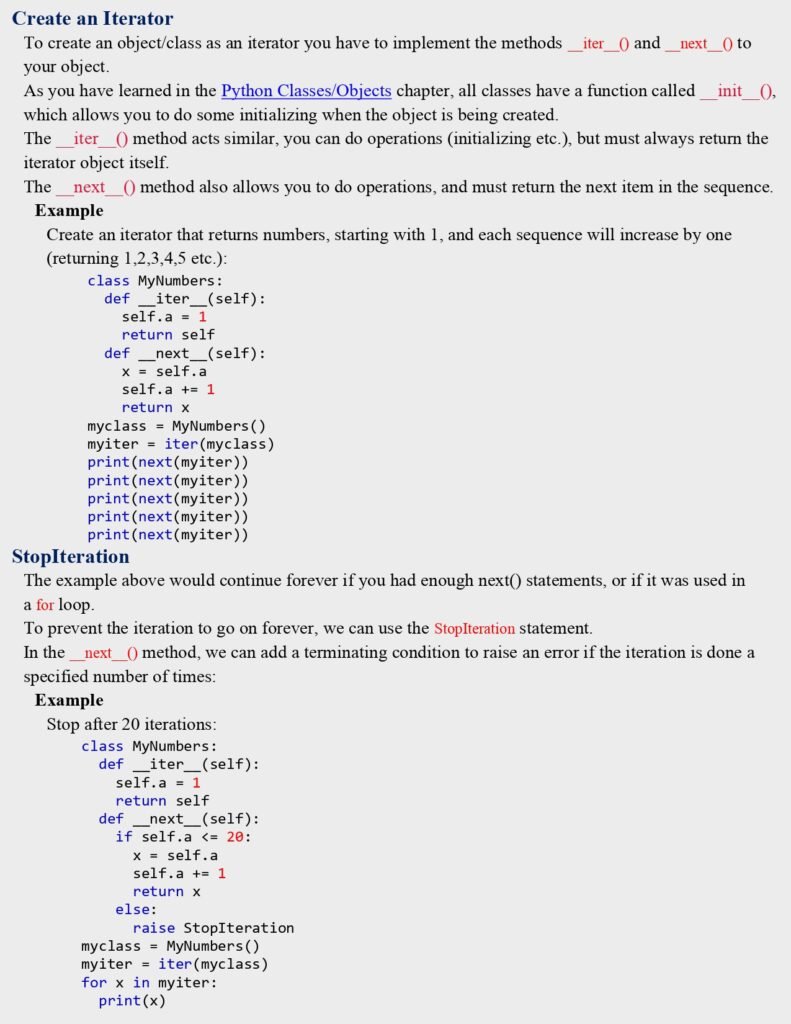
Lesson Content
- Python Inheritance
- Create a Parent Class
- Create a Child Class
- Add the __init__() Function
- Use the super() Function
- Add Properties
- Add Methods
- Python Iterators
- Iterator vs Iterable
- Looping Through an Iterator
- Create an Iterator
- StopIteration
Lesson Format
Nu Of Pages
5
Download Python Lesson 14 From here
Python Lessons
Python From Scratch Lesson 1
Python From Scratch Lesson 2 Pdf (Python Variables)
Python From Scratch Lesson 3 PDF (Python Data Types, Numbers, and Casting)
Python From Scratch Lesson 4 PDF (Python Strings
Python From Scratch Lesson 5 PDF (Python Booleans and Operators)
Python From Scratch Lesson 6 PDF (Python Lists)
Python From Scratch Lesson 7 PDF (Python Tuples)
Python From Scratch Lesson 8 PDF (Python Sets)
Python From Scratch Lesson 9 PDF (Python Dictionaries)
Python From Scratch Lesson 10 PDF (If … Else)
Python From Scratch Lesson 11 PDF (Python While Loops & For Loops)
Python From Scratch Lesson 12 PDF (Python Functions & Lambda)
Python From Scratch Lesson 13 PDF (Python Arrays, Classes and Objects)