Object-Oriented Programming (OOP) is a fundamental paradigm in software development, and Java is a powerful language that fully supports OOP principles. Understanding OOP concepts and their implementation in Java is essential for building robust and scalable applications.
To aid your learning journey, we have created an OOP in Java Full Notes PDF. This comprehensive guide covers all aspects of OOP in Java, providing a detailed and concise resource to deepen your understanding of OOP principles and their practical application in Java programming.
Overview of the PDF Guide
The OOP in Java Full Notes PDF offers an extensive coverage of Object-Oriented Programming concepts and their implementation in Java. It delves into the core principles of OOP, such as encapsulation, inheritance, polymorphism, and abstraction. Additionally, the guide explores advanced topics like interfaces, abstract classes, design patterns, and exception handling. By studying this PDF guide, you’ll gain a solid foundation in OOP and learn how to leverage Java’s features to create well-structured and maintainable code.
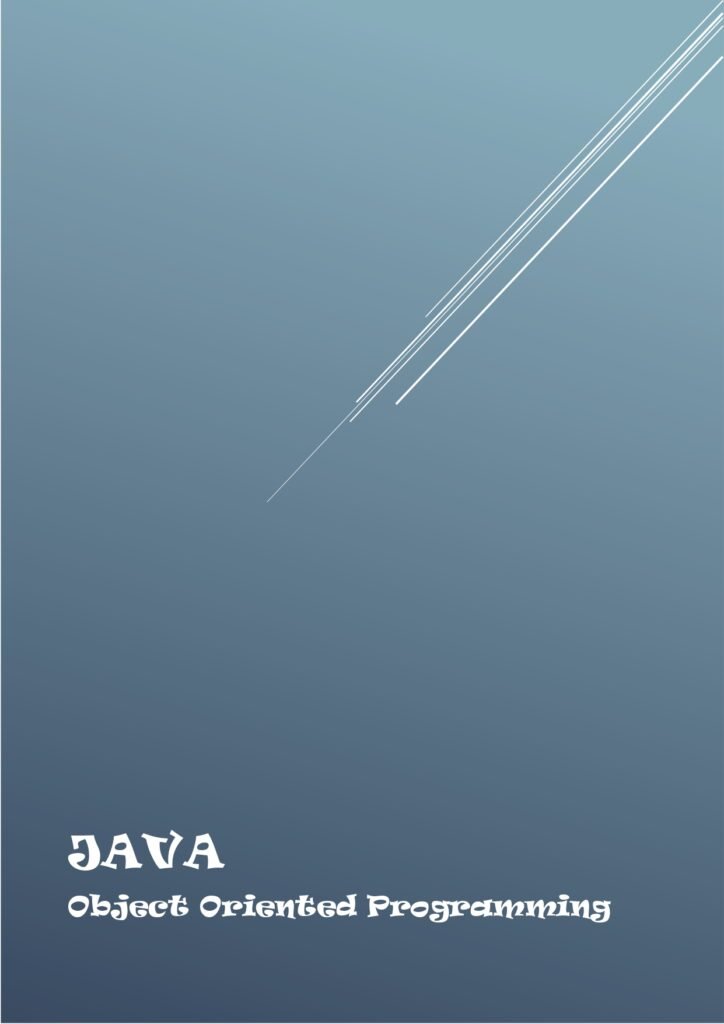
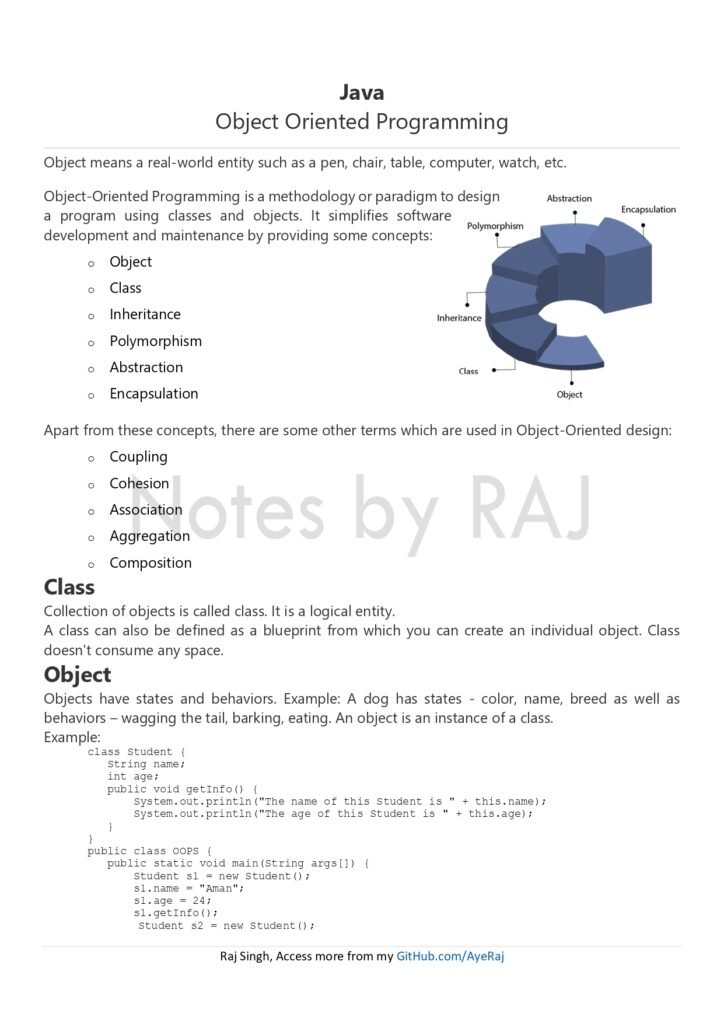
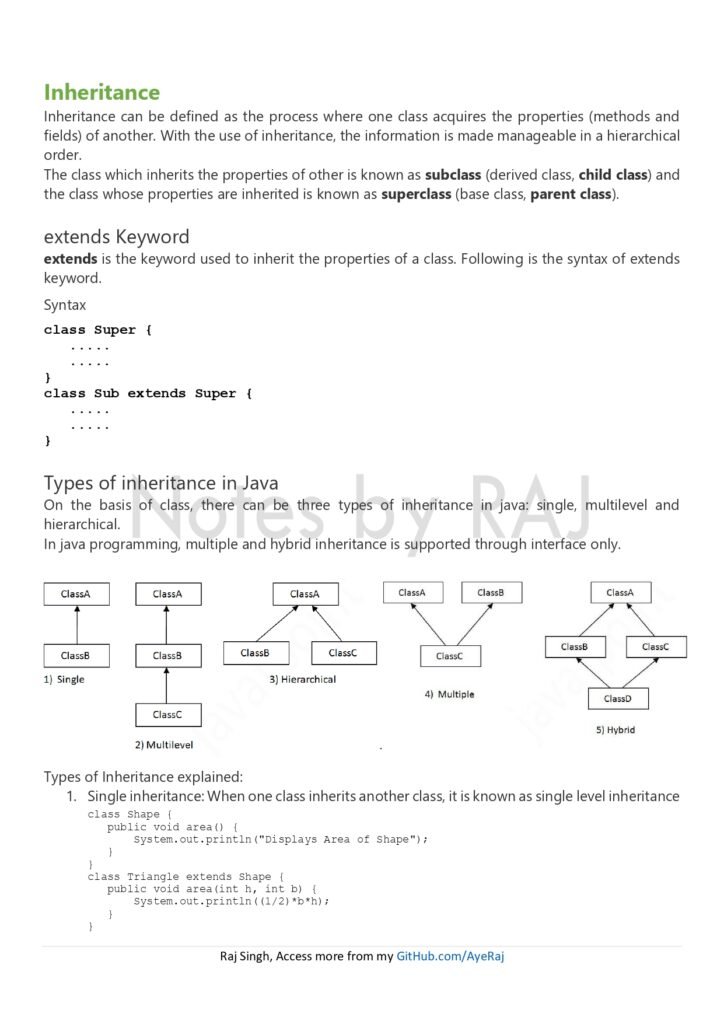
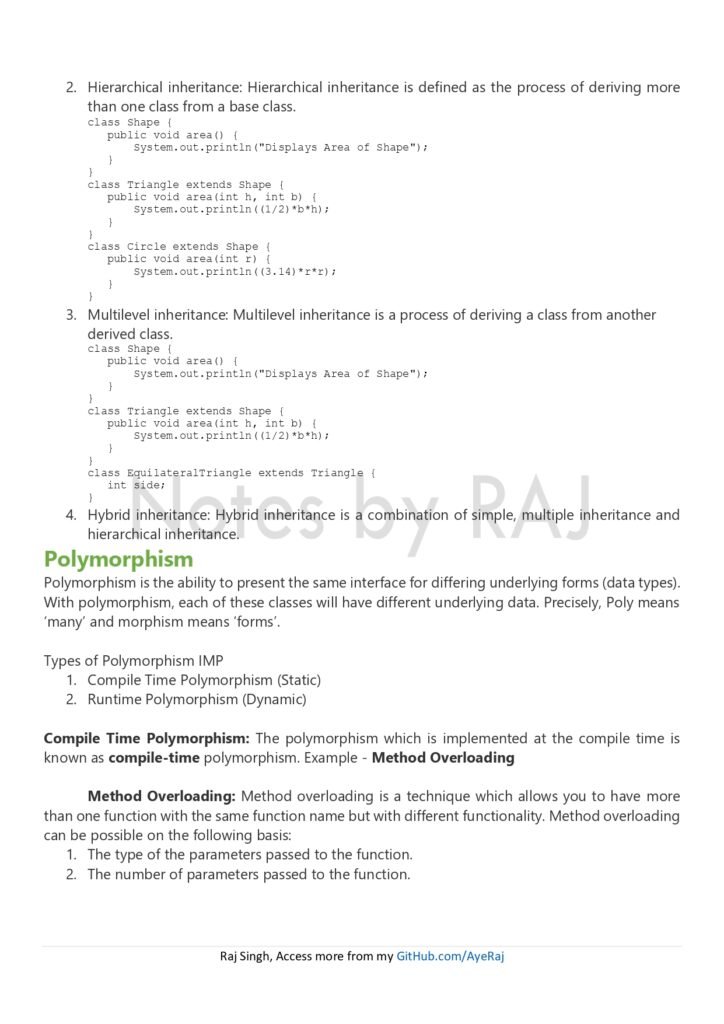
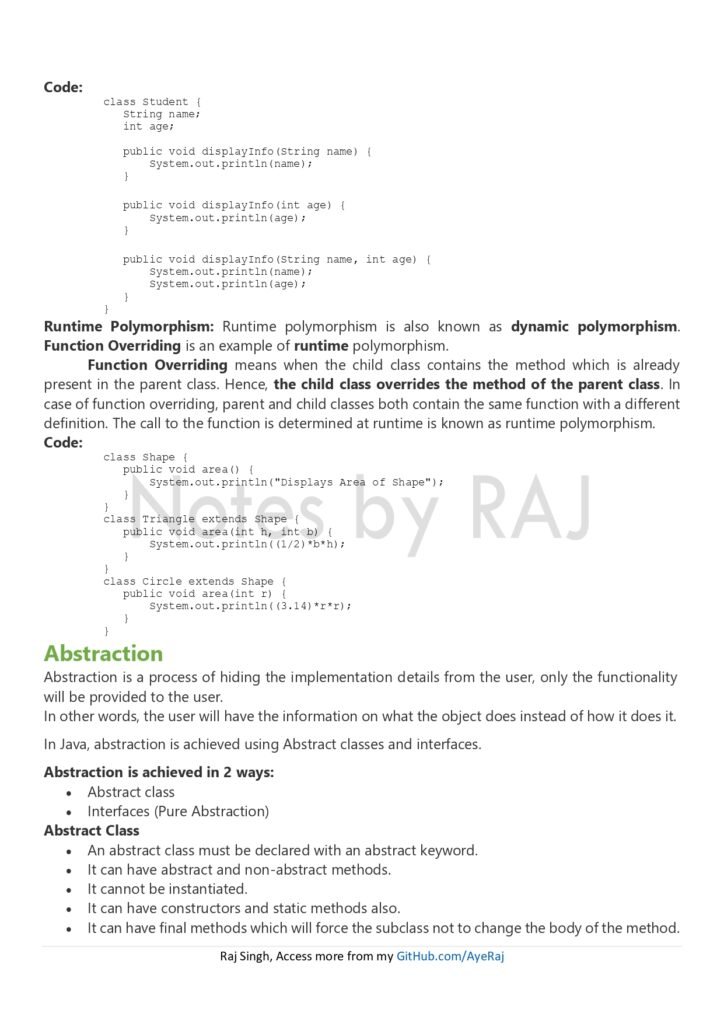
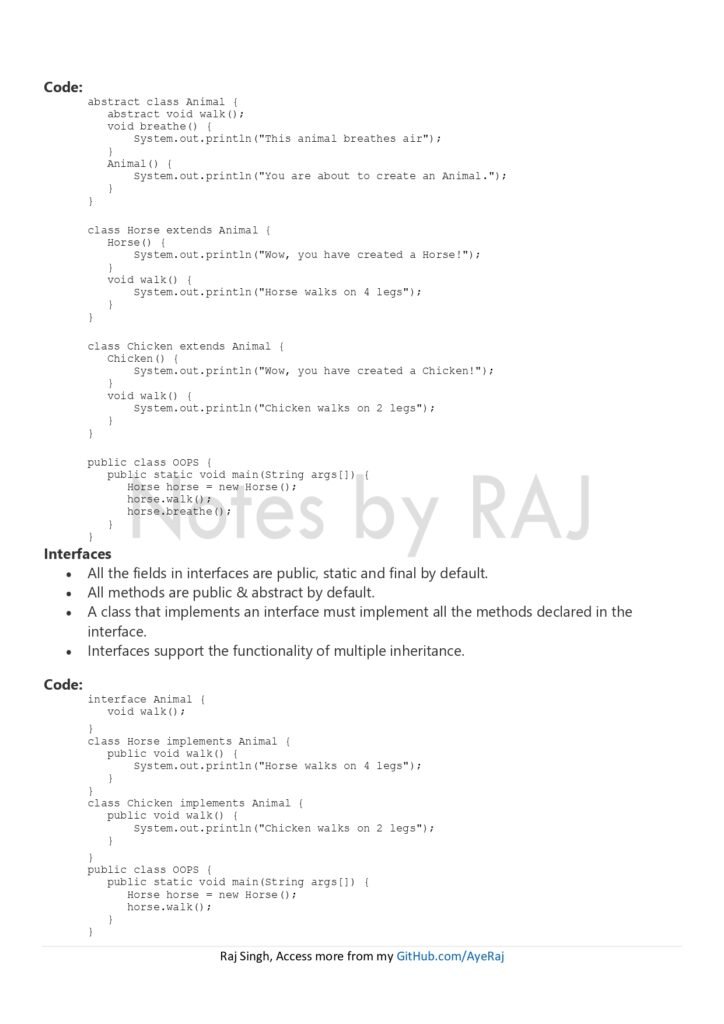
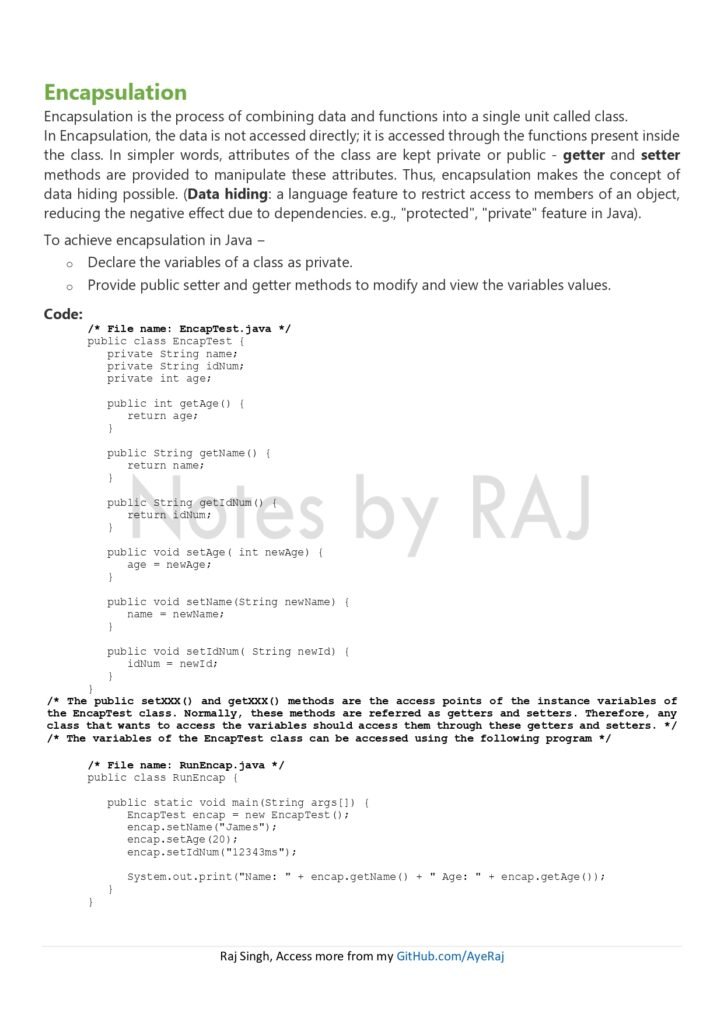
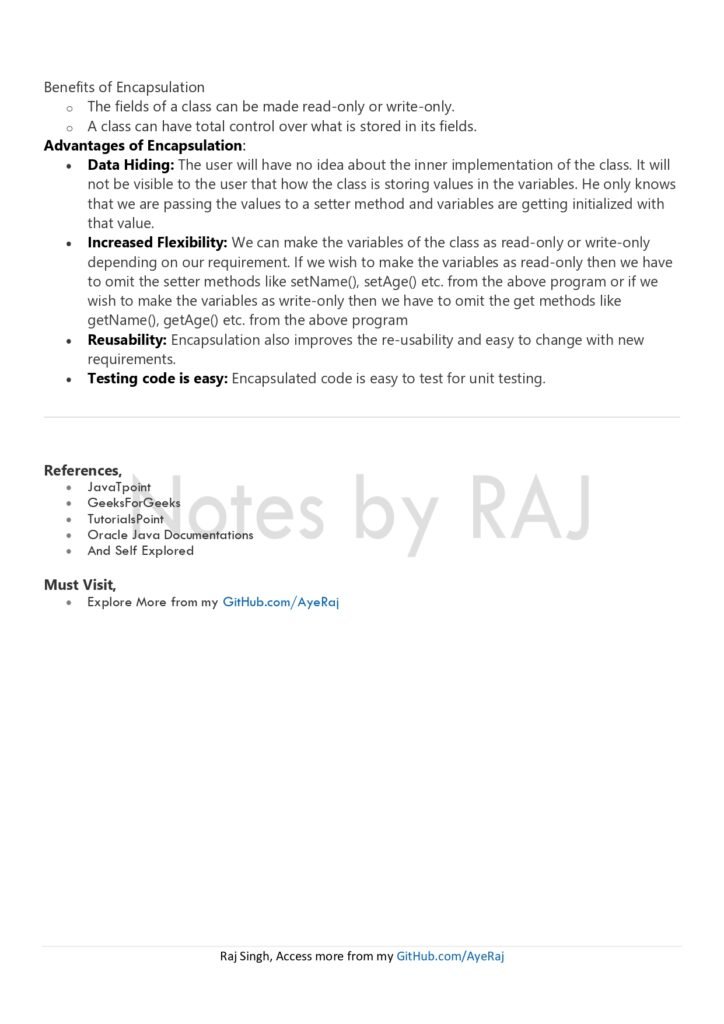
Table of Contents
To provide an overview of the topics covered in the PDF guide, here is a summary of the table of contents:
- Introduction to OOP
- OOP principles and advantages
- Java as an OOP language
- Objects, classes, and methods
- Encapsulation and Access Modifiers
- Access modifiers (public, private, protected)
- Getters and setters
- Data hiding and encapsulation
- Inheritance and Polymorphism
- Inheritance hierarchy and relationships
- Method overriding and dynamic method dispatch
- Polymorphism and its benefits
- Abstraction and Interfaces
- Abstract classes and methods
- Interfaces and their implementation
- Multiple inheritance through interfaces
- Exception Handling
- Handling exceptions in Java
- Try-catch blocks and exception propagation
- Checked and unchecked exceptions
- Design Patterns
- Creational, structural, and behavioral patterns
- Singleton, Factory, Observer, and Strategy patterns
- Applying design patterns in Java
The Benefits of This Guide
The OOP in Java Full Notes PDF offers several benefits to learners:
- Comprehensive Coverage: The guide covers all essential OOP concepts used in Java programming. From the fundamentals of objects and classes to advanced topics like inheritance, interfaces, and design patterns, you’ll gain a holistic understanding of OOP in Java.
- Detailed Explanations: Each concept is explained in detail, providing clear explanations, examples, and code snippets to illustrate the implementation. This enables you to grasp the concepts thoroughly and apply them effectively in your own Java projects.
- Practical Application: The guide emphasizes practical application by discussing real-world scenarios where OOP concepts are commonly used. This helps you understand how OOP principles can be applied to solve complex programming problems and build scalable software solutions.
- Reference Guide: The PDF format allows you to easily access and navigate the guide, making it a handy reference resource during your Java programming journey. You can refer back to specific topics, refresh your knowledge, and reinforce your understanding of OOP in Java.
Conclusion
The OOP in Java Full Notes PDF is an invaluable resource for anyone seeking a comprehensive understanding of Object-Oriented Programming and its implementation in Java. By studying this guide, you’ll gain a solid foundation in OOP principles and learn how to leverage Java’s features to write clean, modular, and maintainable code. Embrace this PDF guide as a tool to enhance your Java programming skills and unlock the power of OOP in your software development endeavors. Happy coding!
Nu Of Pages:
8 Pages
Download the OOP in Java Full Notes PDF from here
For Java Tutorial PDF From here