In this blog post, I will share with you a PDF document that contains notes on arrays in Java. An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created, and cannot be changed later.
Arrays are one of the most commonly used data structures in Java, as they allow us to store and manipulate multiple values efficiently and conveniently.
Overview of the Notes
The PDF document that I have prepared for you consists of 14 pages, divided into six sections. The first section is an introduction to array, where you will learn the general idea and purpose of array, as well as the common terminology and syntax.
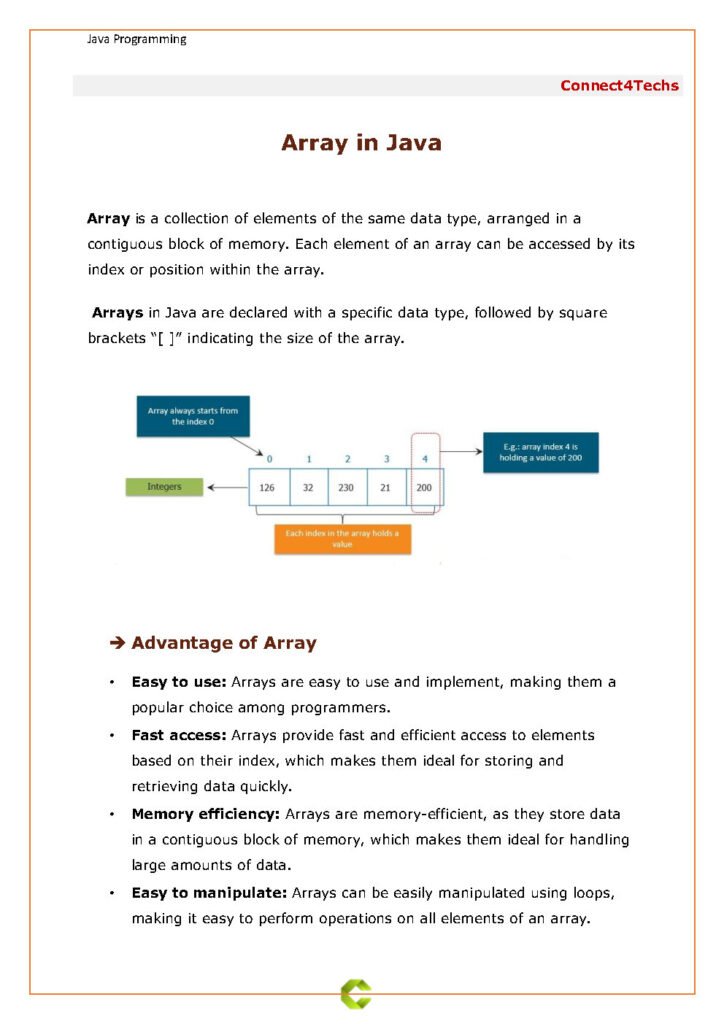
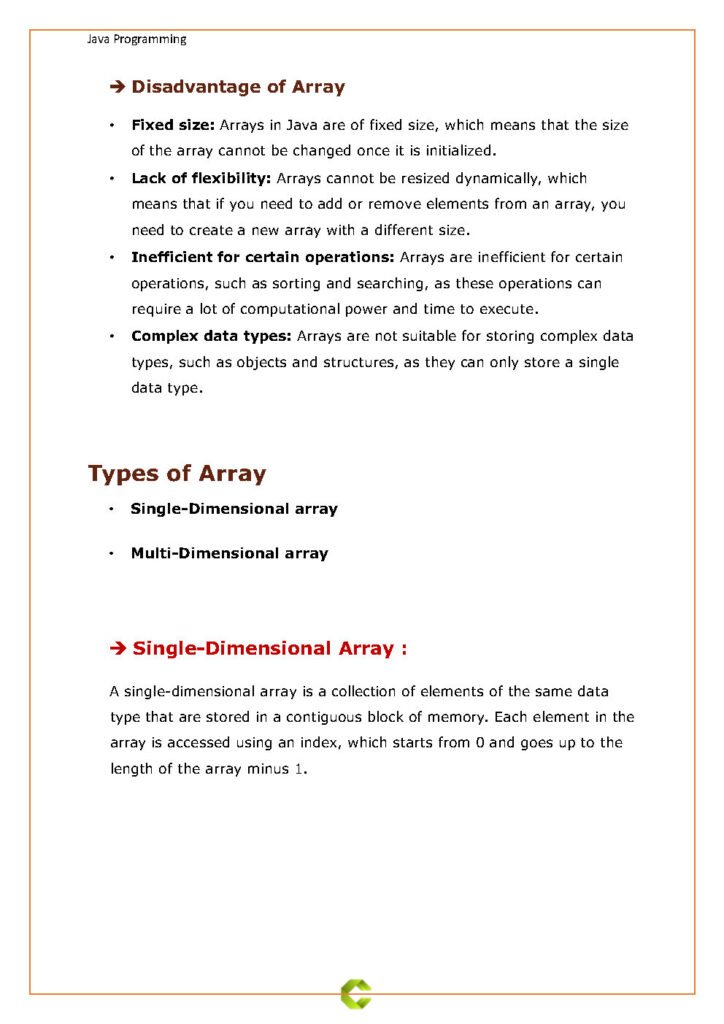
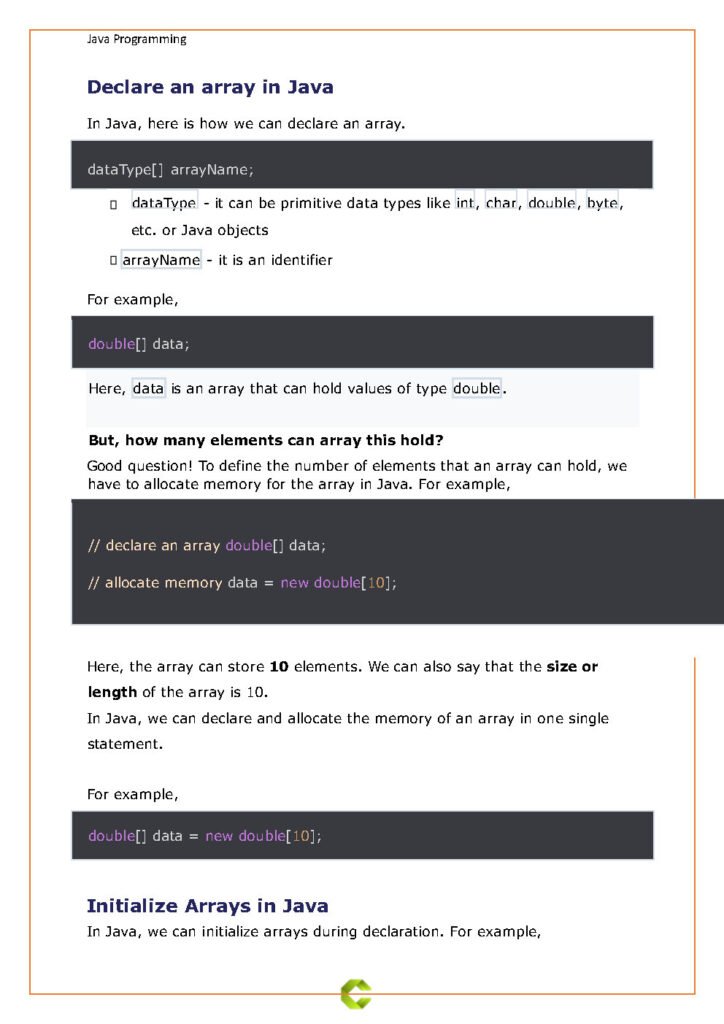
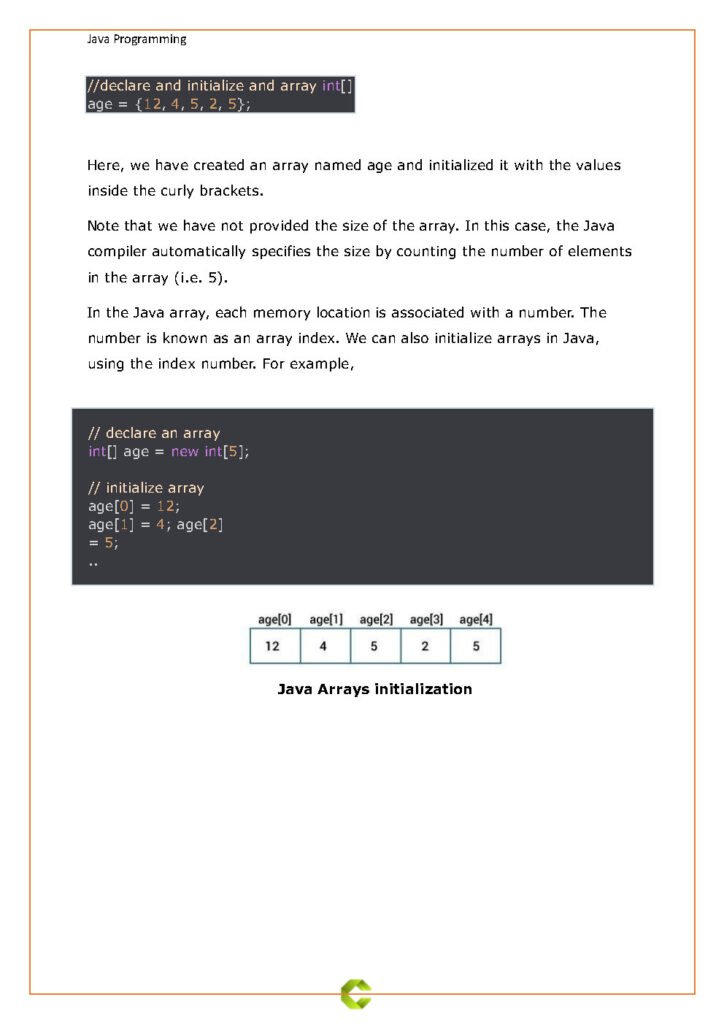
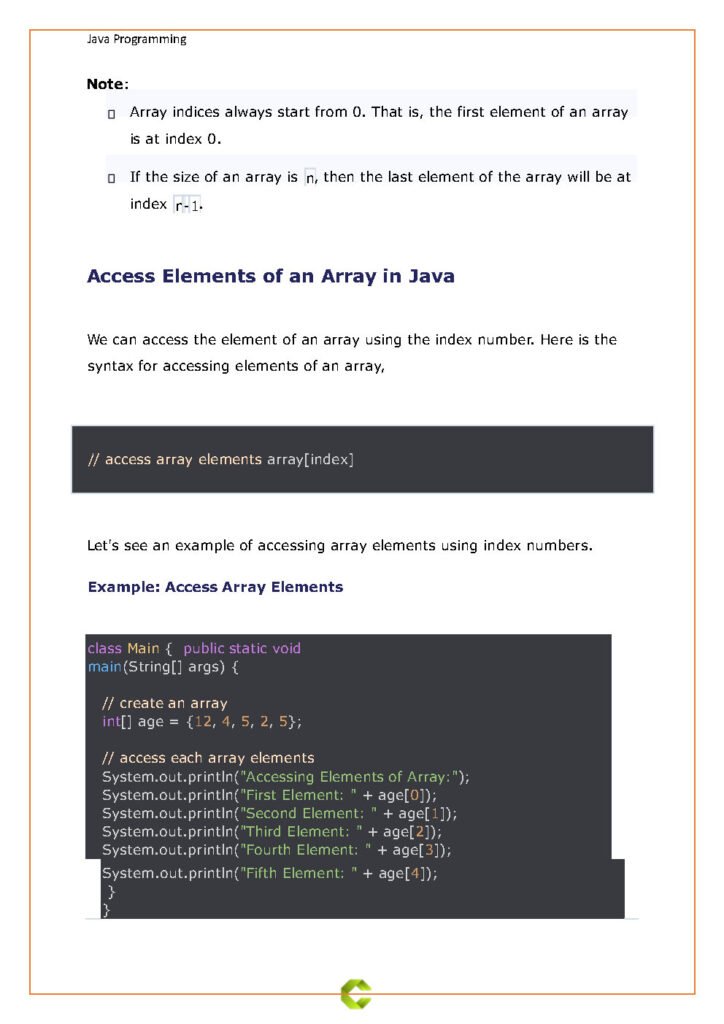
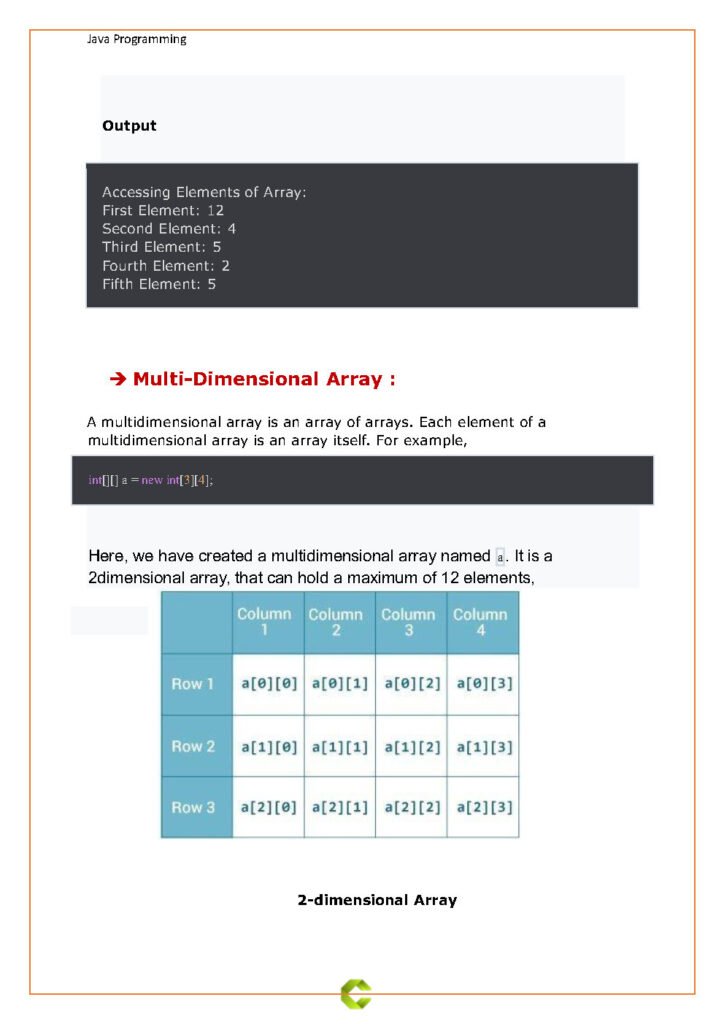
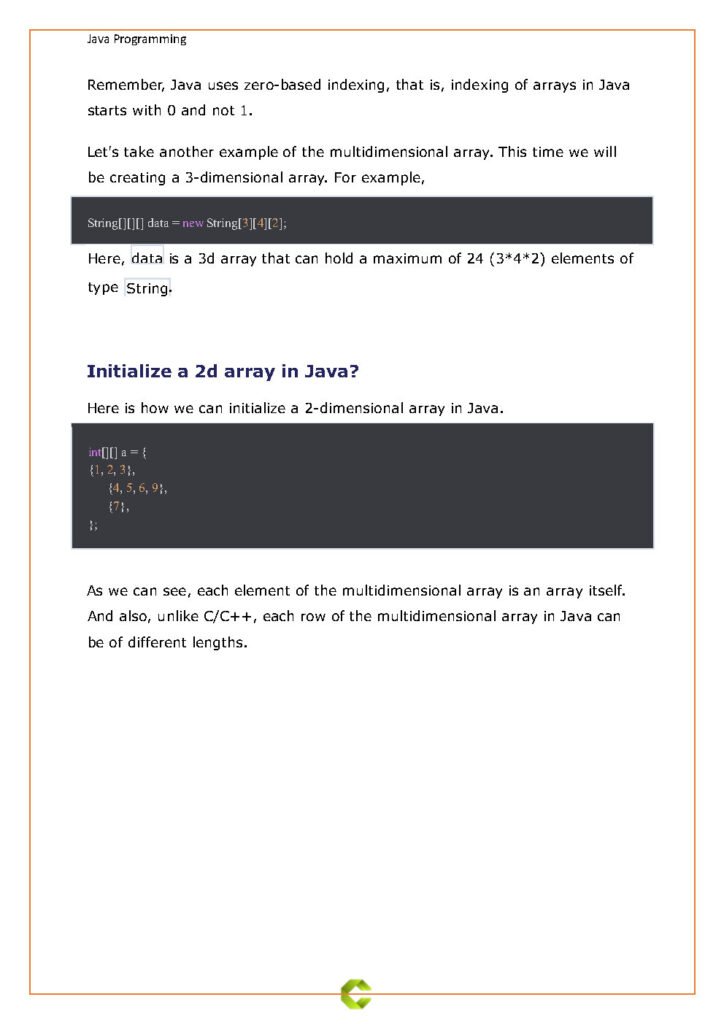
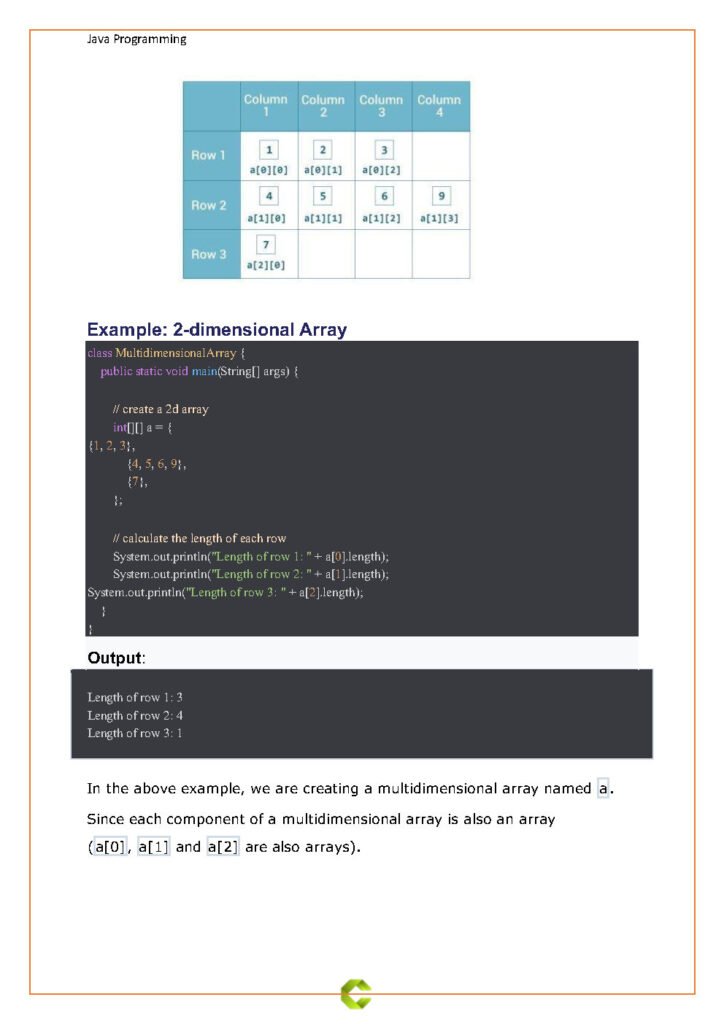
The second section is a detailed explanation of how to declare and initialize arrays in Java, where you will learn the different ways to create and assign values to arrays. The third section is a detailed explanation of how to access and modify array elements in Java, where you will learn how to use the index number and the for loop to manipulate array values. The fourth section is a detailed explanation of how to use multidimensional arrays in Java, where you will learn how to create and use arrays of arrays, such as two-dimensional and three-dimensional arrays. The fifth section is a detailed explanation of how to use some built-in methods and classes for array operations in Java, where you will learn how to use the Arrays and ArrayList classes, as well as the System.arraycopy and Arrays.copyOf methods. The sixth section is a summary and a quiz, where you will review the main points and test your understanding with some exercises.
The Content of the Notes
The PDF document that I have prepared for you contains the following content:
- Introduction to Array
- What is an array?
- Why do we need arrays?
- What are the characteristics of arrays in Java?
- How to declare an array in Java?
- Declaring and Initializing Arrays in Java
- How to create an array using the new operator?
- How to create an array using the literal notation?
- How to create an array using the anonymous array syntax?
- How to assign values to array elements?
- Accessing and Modifying Array Elements in Java
- How to access array elements using the index number?
- How to modify array elements using the index number?
- How to use the for loop to iterate over array elements?
- How to use the enhanced for loop to iterate over array elements?
- Multidimensional Arrays in Java
- What is a multidimensional array?
- How to create a multidimensional array in Java?
- How to access and modify multidimensional array elements in Java?
- How to use nested loops to iterate over multidimensional array elements in Java?
- Built-in Methods and Classes for Array Operations in Java
- How to use the Arrays class to perform various array operations?
- How to use the ArrayList class to create dynamic arrays?
- How to use the System.arraycopy method to copy array elements?
- How to use the Arrays.copyOf method to copy and resize arrays?
- Summary and Quiz
- Key points to remember
- Multiple choice questions
- Fill in the blanks questions
- Coding challenges
Why This Notes
This PDF document is a useful resource for anyone who wants to learn or review the basics of array in Java. It covers the most important and common aspects of array, with clear explanations and examples. It also provides some exercises to practice and reinforce your learning. By reading this PDF, you will be able to:
- Understand the concept and purpose of array
- Write and execute different types of array in Java
- Use array to store and manipulate multiple values
- Avoid common errors and pitfalls when using array
Conclusion
I hope you find this PDF document helpful and informative. Array is an essential part of programming, and mastering it will make you a better and more efficient programmer. If you have any questions or feedback, please feel free to leave a comment below. Thank you for reading and happy coding!
Download from Link
You can download the PDF document from this link: Array in Java PDF.